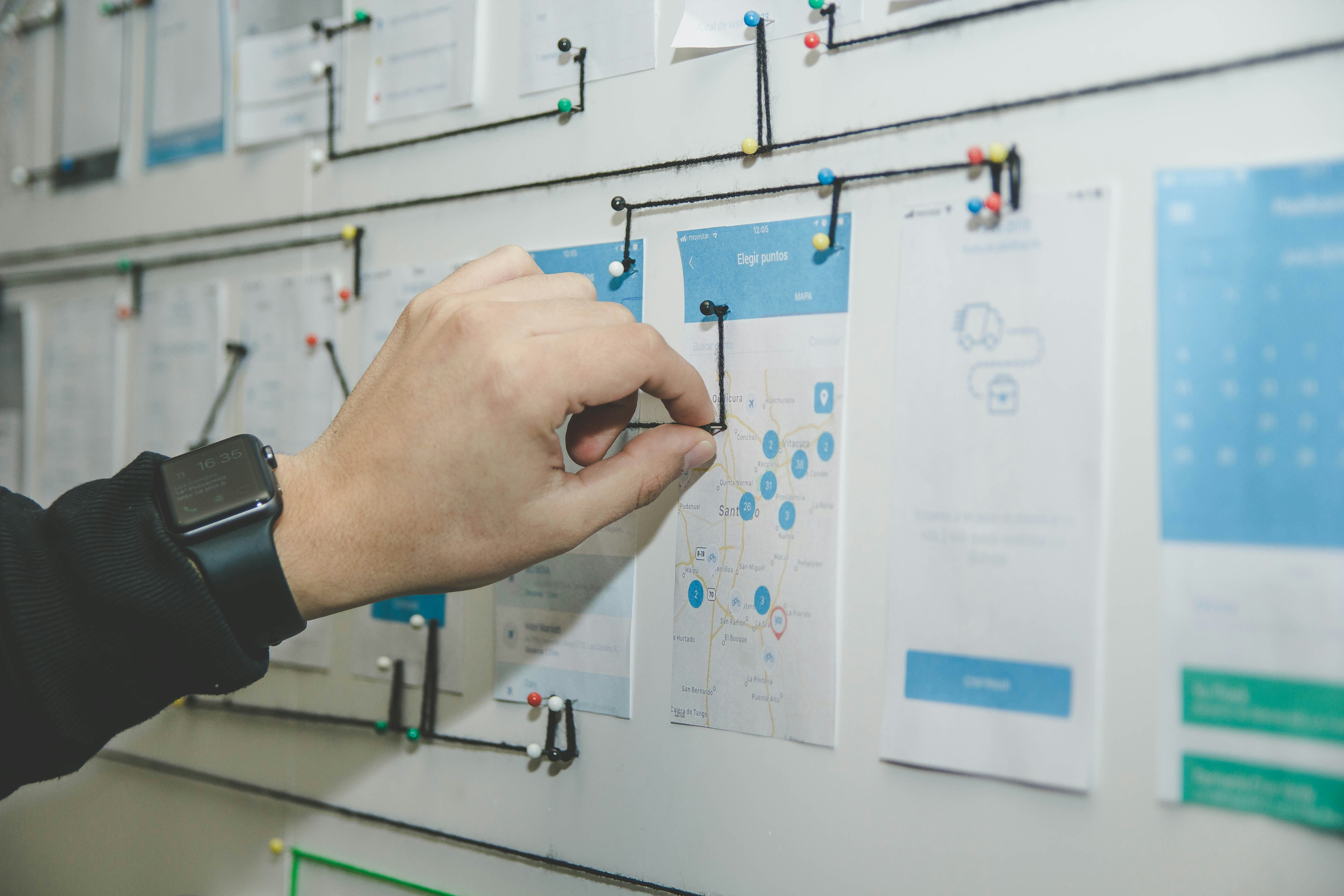
This is a note for describing ui from react.dev. ive added some more points and its explanation and links
Components
Components, reusable UI elements for your app, which contain html,css,js. React component is a JavaScript function that you can sprinkle with markup. Declare components in the top level.
import WieredVillans from './WieredVillans'; <-- this is an import for default export
function Profile() {
return (
<img
src="https://i.imgur.com/MK3eW3As.jpg"
alt="Katherine Johnson"
/>
);
}
export default function Gallery() { <--can be default export like this
return (
<section>
<Profile />
<WieredVillans />
</section>
);
}
## imagine that below code is another file named BookGallry.jsx
import { Button } from './Button.js'; <-- this is an import for named export
export function BookGallery() { <--can be named export like this
return (
<section>
<Button />
</section>
);
}
JSX
JSX is a special way to write code that looks like HTML but works inside JavaScript, making it easier to create web pages with React.
React, rendering logic and markup live together in the same place components.
can use jsx seperately
Rules of jsx
- JSX Must Have One Parent Element
- JSX Tags Must Be Closed
- JSX Attributes Use CamelCase
- JavaScript Expressions in JSX
- CSS in JSX
- Comments in JSX
- JSX Prevents Injection Attacks
- JSX Elements are Immutable
Usage of {} in jsx
- Embedding JavaScript Expressions
<h1>Hello, {name}!</h1>;
- Dynamic Attributes
<img src={imageUrl} alt="Example" />;
- Conditional Rendering
<div>{isLoggedIn ? 'Welcome back!' : 'Please log in.'}</div>;
- Inline Styles
<div style={style}>Styled Text</div>;
- Rendering Arrays
const items = ['Apple', 'Banana', 'Cherry'];
return (
<ul>
{items.map(item => (
<li key={item}>{item}</li>
))}
</ul>
);
props
Props are the information that you pass to a JSX tag.
<Avatar
person={{ name: 'Lin Lanying', imageId: '1bX5QH6' }}
size={100}
/>
Destructuring
# here default size is 100
function Avatar({ person, size=100 }) {
// ...
}
function Avatar(props) {
let person = props.person;
let size = props.size;
// ...
}
Forwarding props with the JSX spread syntax
function Profile(props) {
return (
<div className="card">
<Avatar {...props} /> <-- its "spread"
</div>
);
}
Important points on props
- Props Can Change Over Time .
- Props are Immutable: Props cannot be changed directly. To change props, a component must ask its parent to pass different props.
- Use State for User Input: To respond to user input, use state instead of trying to change props
conditional rendering
- using if
if (isPacked) { '✅' }
- using Conditional (ternary) operator (? :)
{isPacked ? '✅' : '' }
- using Logical AND operator (&&)
{isPacked && '✅'}
. A JavaScript && expression returns the value of its right side (in our case, the checkmark) if the left side (our condition) is true.
rendering lists
- use filter() and map() with React to filter and transform your array of data into an array of components.
- use key to list items in order
More on key
- Keys tell React which array item each component corresponds to (or uniquely identify an item between its siblings ), so that it can match them up later. This becomes important if your array items can move (e.g. due to sorting), get inserted, or get deleted. A well-chosen key helps React infer what exactly has happened, and make the correct updates to the DOM tree.
- use crypto.randomUUID() or a package like uuid to generate key or take it from back end
- Dont use loop index as keys
- Dont create random keys on the fly
Keep components pure
- React assumes that every component you write is a pure function (ie, no sode effects).
- we can mutate data we create inside our component , Local mutation
- side effects - changing anything in a components - happens inside event handlers. These are not run during so not need to be pure.
Why some components breaks ?
we can use strict mode [no effect in production] in which it calls each component’s function twice during development. By calling the component functions twice, Strict Mode helps find components that break these rules. so keep components pure.
Why pure ?
- react can skip rendering
- can start and stop rendering without worrying about side effects.
React’s Tree
- Rendering
- React also uses tree structures to manage and model the relationship between components in a React app. These trees are useful tools to understand how data flows through a React app and how to optimize rendering and app size.
- render tree is only composed of React components.
- Conditional rendering
- Shows where components are rendered
- Module
- non component modules will be included in this tree
- builder - tool responsible to bundle all these js
- understanding of bundling will help us to fix perfomance issues